Adding zoned background music
Creating a simple ambiant audio toggle with multiple zones.
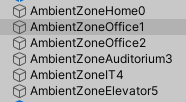
First create your zones by creating new empty game objects in the inspector for each zone.
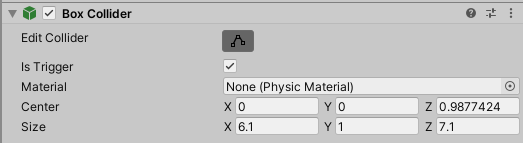
Add a collider to each and make sure to set the checkbox "Is Trigger" because the script is looking for a trigger and not an actual collision.. Box collider works well for squares but spheres and capsule will work as well.
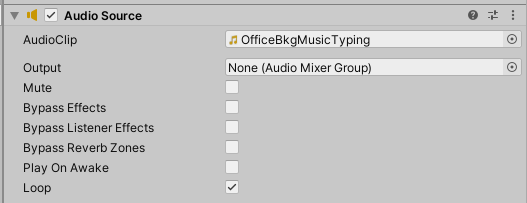
Add an Audio Source to each new empty zone game object and add the sound file you want the area to play as your audio clip. Make sure that Loop is checked so that it plays the entire time you are "in the zone".
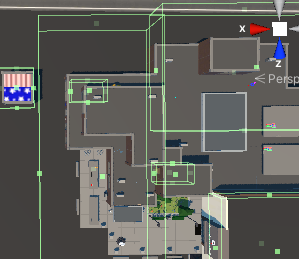
Drag each zone game object to the location you want the audio to play and resize the collider (using the Edit Collider button on the collider) to the size of this zone area.
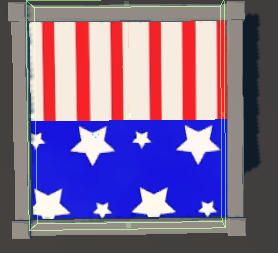
Collider height may or may not come into play depending on your game. To view zones together for overlaps, select all the zones in the hierarchy (shift-click or control-click) while the box collider view is open.
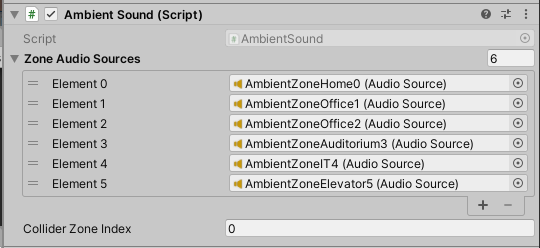
Create the script using one of the zones or paste it into one of the asset folders unity and add it to the empty game object.
Increase the audio zone list by adding a numeric value of the number of zones you will have. (6 in this example) You should now see 6 audio source slots to drag your zones into from the left hierarchy list. You will need to repeat this for reach zone. The reason for having all is that the first collision action is to turn all the zones off and only turn on the zone set for that game object.
For each zone game object script, set the Collider Zone Index to match the Audio Source Element # (element 0, element 1) audio that you want that zone to play.
The script example watches for collisions from objects that have the "Player" tag. If your players have a different tag then change that to match.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/* Audio zone trigger script
Turns on and off zone audio on triggering onenter and onexit.
abitowhit 5/25/2022 https://247coding.com
*/
public class AmbientSound : MonoBehaviour
{
[SerializeField]
AudioSource[] zoneAudioSources;
[SerializeField]
int colliderZoneIndex=-1;
int curZoneIndex=-1;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
void SoundReset()
{
foreach (AudioSource src in zoneAudioSources)
{
src.Stop();
}
}
void OnTriggerEnter(Collider col)
{
if(col.gameObject.tag=="Player")
{
if(colliderZoneIndex > -1 && colliderZoneIndex < zoneAudioSources.Length && curZoneIndex != colliderZoneIndex)
{
SoundReset();
curZoneIndex = colliderZoneIndex;
((AudioSource)zoneAudioSources[colliderZoneIndex]).Play();
}
}
}
void OnTriggerExit(Collider col)
{
if(col.gameObject.tag=="Player")
{
SoundReset();
}
}
}