Submitted by abitowhit on Thu, 05/16/2024 - 19:01
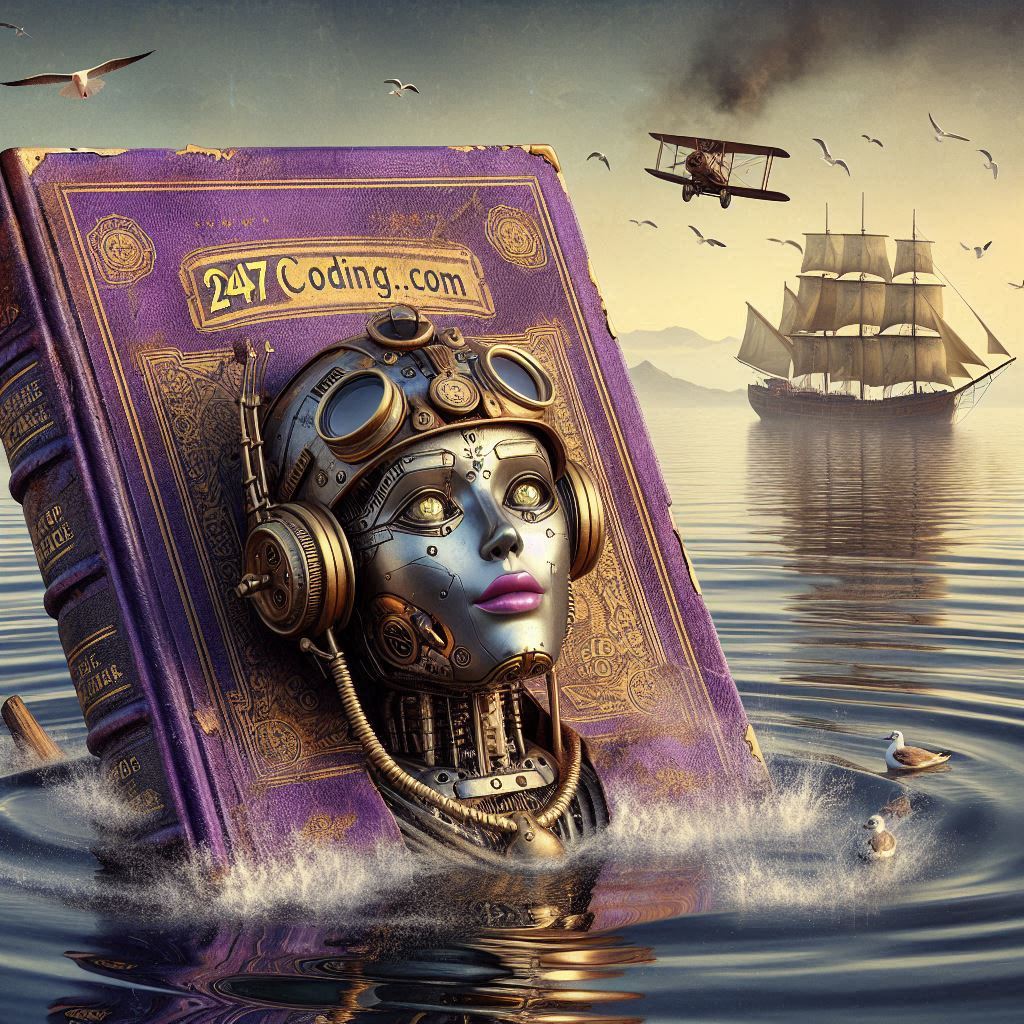
Playing with copilot last night and I will have to admit, I was amused andsomewhat impressed.
Cool stuff. Basically a placeholder for an upcoming project I am looking into.
Noted:
This code is not tested, Not verified, Not yet reviewed, it was just a sample attempt created using copilot for testing AI suggested code for evaluation on a possible new project.
AI generated C# simple sample BT class for wifi
using Windows.Devices.Radios;
using Windows.Devices.Bluetooth;
using Windows.Devices.Enumeration;
using System.Threading.Tasks;
public class BlueManager
{
private async Task GetBluetoothRadioAsync()
{
var radios = await Radio.GetRadiosAsync();
foreach (var radio in radios)
{
if (radio.Kind == RadioKind.Bluetooth)
{
return radio;
}
}
return null;
}
private async Task GetWifiRadioAsync()
{
var radios = await Radio.GetRadiosAsync();
foreach (var radio in radios)
{
if (radio.Kind == RadioKind.WiFi)
{
return radio;
}
}
return null;
}
public async Task TurnOffWifiIfBluetoothDeviceNotNearby(string bluetoothDeviceId)
{
var bluetoothRadio = await GetBluetoothRadioAsync();
var wifiRadio = await GetWifiRadioAsync();
if (bluetoothRadio != null && wifiRadio != null)
{
var deviceWatcher = DeviceInformation.CreateWatcher(
BluetoothDevice.GetDeviceSelectorFromPairingState(true));
deviceWatcher.Added += (sender, args) =>
{
if (args.Id == bluetoothDeviceId)
{
// The specific Bluetooth device is nearby
// Do nothing or turn on Wi-Fi if it's off
}
};
deviceWatcher.Removed += async (sender, args) =>
{
if (args.Id == bluetoothDeviceId)
{
// The specific Bluetooth device is not nearby
// Turn off Wi-Fi
await wifiRadio.SetStateAsync(RadioState.Off);
}
};
deviceWatcher.Start();
}
}
}